Python Modules and Packages: A Comprehensive Guide
Python is one of the most versatile programming languages available today. Whether you’re developing web applications, handling data science projects, or automating tasks, Python provides a rich ecosystem of modules and packages to simplify your workflow. Understanding how to use modules and packages effectively can significantly improve your code structure, maintainability, and reusability.
In this blog, we’ll delve into Python modules and packages, covering their importance, how to create and use them, and best practices for organizing your Python projects efficiently.
What Are Python Modules?
A module in Python is simply a file containing Python code. Modules allow us to logically organize our Python code into separate files, making it easier to manage and reuse. A module can define functions, classes, and variables, and it may include runnable code as well.
How to Create a Python Module
Creating a module is as simple as writing Python code in a .py
file. For example, let’s create a module named math_operations.py
:
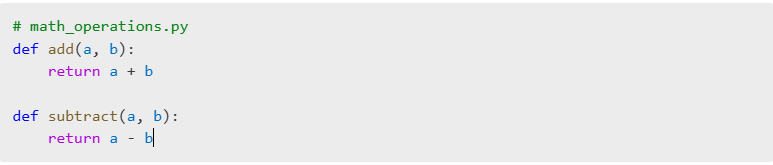
Now, you can use this module in another Python script by importing it:

Alternatively, you can import specific functions:

Built-in Modules in Python
Python comes with a large number of built-in modules that provide various functionalities without the need for external installation. Some commonly used built-in modules include:
math
: Provides mathematical functions likesqrt
,pow
,ceil
, etc.os
: Enables interaction with the operating system.sys
: Provides system-specific parameters and functions.random
: Allows random number generation.datetime
: Helps in working with dates and times.
Example usage:

What Are Python Packages?
A package is a way of organizing related Python modules into a directory hierarchy. It helps manage large codebases by structuring modules logically.
A package is simply a directory containing:
- One or more module files (
.py
files) - A special
__init__.py
file (which can be empty or contain package initialization code)
How to Create a Python Package
Let’s create a package called my_package
containing multiple modules:

operations.py
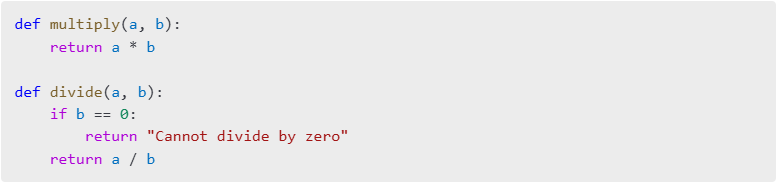
greetings.py

Using the Package
To use this package in your project:

Alternatively, import specific functions:

Installing and Using External Packages
While Python’s standard library is extensive, sometimes you need third-party packages. These can be installed using pip, Python’s package manager.
Installing a Package with Pip
To install a package:
pip install requests
Using the installed package:

To list installed packages:
pip list
To uninstall a package:
pip uninstall requests
Best Practices for Using Modules and Packages
Keep Modules Small and Focused: Each module should serve a specific purpose rather than containing unrelated functionalities.
Use Meaningful Names: Choose descriptive names for modules and packages.
Avoid Circular Imports: Circular dependencies can cause import errors. Structure modules properly to prevent such issues.
Use
__init__.py
Wisely: Keep__init__.py
minimal or use it to expose only the necessary components of the package.Follow a Directory Structure: Organize your code effectively, especially in large projects. A common project structure is:
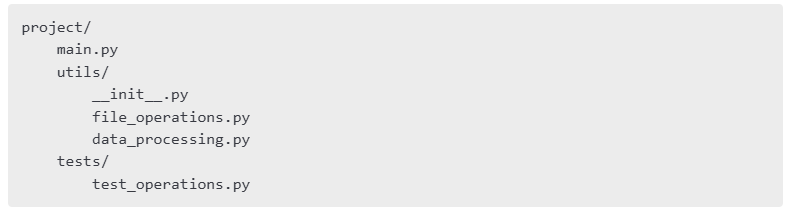
Conclusion
Modules and packages are fundamental to writing clean, maintainable Python code. By properly organizing your project into modules and packages, you can improve code reuse, readability, and scalability. Whether you’re using built-in modules, creating your own, or installing third-party packages, mastering these concepts will make you a more efficient Python developer.