Understanding Python Loops: For vs. While
Loops are an essential feature of programming, enabling you to execute a block of code multiple times efficiently. In Python, the two primary types of loops are for
** loops** and while
** loops**. While they serve similar purposes, they are best suited to different scenarios. In this article, we’ll explore how these loops work, when to use them, and their key differences.
The for Loop
A for
loop is used when you know the number of iterations beforehand or need to iterate through a sequence like a list, tuple, string, or range.
Syntax

Example: Iterating Over a Range

Example: Iterating Over a List

The for
loop makes it easy to traverse elements of a sequence or iterate a known number of times.
The while Loop
A while
loop is used when the number of iterations is not known in advance, and you need to repeat the block of code as long as a specific condition is true.
Syntax

Example: Count Until a Condition is Met

With while
, the condition is checked before each iteration, making it ideal for scenarios where the stopping point is dynamic.
Key Differences Between for and while Loops
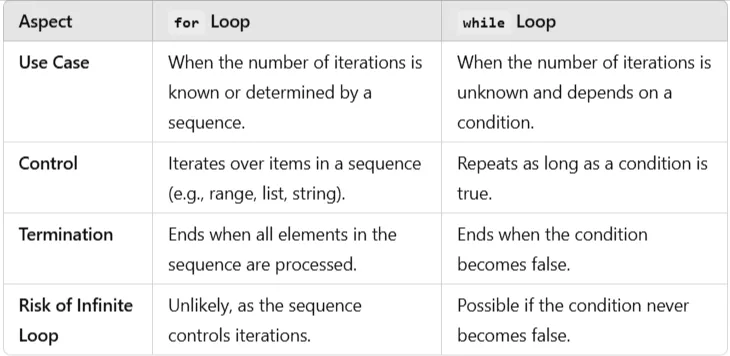
When to Use Each Loop
Use for Loop When:
- You are iterating over elements of a sequence.
- The number of iterations is predefined.
- Traversing through items like numbers, strings, or lists.
Use while Loop When:
- You don’t know how many iterations are needed.
- The loop depends on a condition being met at runtime.
- You’re working with dynamic stop conditions.
Common Pitfalls
Infinite Loops in while
: Be cautious with conditions in while
loops. An infinite loop can occur if the condition never evaluates to False
. Always ensure the loop has a clear exit.
Off-by-One Errors in for
: Pay attention to the range. For example, range(5)
generates numbers 0 through 4, not 1 through 5.
Conclusion
Both for
and while
loops are powerful tools for iteration in Python. Choosing the right loop depends on the problem:
- Use a
for
loop when iterating over a known sequence or range. - Use a
while
loop when the stopping condition is dynamic or uncertain.